spring boot 에서 mybatis 를 연동하는 법을 해보자
pom.xml
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
일단 pom.xml 에 mybatis 라이브러리부터 추가하자. dbms 에 맞는 driver 는 추가됐다고 가정하고 진행한다.
사용버젼 표
아래 표에 맞게 version 명시하면 된다.
MyBatis-Spring-Boot-Starter | MyBatis-Spring | Spring Boot | Java |
2.2 | 2.0 (need 2.0.6+ for enable all features) | 2.5 or higher | 8 or higher |
2.1 | 2.0 (need 2.0.6+ for enable all features) | 2.1 - 2.4 | 8 or higher |
1.3 | 1.3 | 1.5 | 6 or higher |
spring boot 2.5 이상부터 mybatis-spring-boot-starter 2.2 버젼이 사용가능하다.
config 클래스
@Configuration
@MapperScan(value="com.juntcom.api.mapper.*", sqlSessionFactoryRef="dbMybatisSqlSessionFactory")
@Slf4j
public class DbMybatisConfig {
@Autowired
private Environment env;
private static final String prefix = "spring.db.datasource.hikari.";
@Bean(name = "dbMybatisSource", destroyMethod = "close")
@Primary
public HikariDataSource dbMybatisSource() {
HikariConfig config = new HikariConfig();
config.setUsername(env.getProperty(prefix+"username"));
config.setPassword(env.getProperty(prefix+"password"));
// config.setDriverClassName(env.getProperty(prefix+"driverClassName"));
config.setJdbcUrl( env.getProperty(prefix+"jdbc-url") );
config.setMaxLifetime( Long.parseLong(env.getProperty(prefix+"max-lifetime")) );
config.setConnectionTimeout(Long.parseLong( env.getProperty(prefix+"connection-timeout")));
config.setValidationTimeout(Long.parseLong( env.getProperty(prefix+"validation-timeout")));
config.addDataSourceProperty( "cachePrepStmts" , env.getProperty(prefix+"data-source-properties.cachePrepStmts"));
config.addDataSourceProperty( "prepStmtCacheSize" , env.getProperty(prefix+"data-source-properties.prepStmtCacheSize"));
config.addDataSourceProperty( "prepStmtCacheSqlLimit" , env.getProperty(prefix+"data-source-properties.prepStmtCacheSqlLimit") );
config.addDataSourceProperty( "useServerPrepStmts" , env.getProperty(prefix+"data-source-properties.useServerPrepStmts") );
HikariDataSource dataSource = new HikariDataSource( config );
return dataSource;
}
@Bean(name = "dbMybatisSqlSessionFactory")
@Primary
public SqlSessionFactory dbMybatisSqlSessionFactory(@Qualifier("dbMybatisSource") DataSource dbMybatisSource) throws Exception {
SqlSessionFactoryBean sqlSessionFactoryBean = new SqlSessionFactoryBean();
sqlSessionFactoryBean.setDataSource(dbMybatisSource);
// sqlSessionFactoryBean.setMapperLocations(new PathMatchingResourcePatternResolver().getResources("classpath:sql/read/*.xml"));
sqlSessionFactoryBean.setMapperLocations(new PathMatchingResourcePatternResolver().getResources("classpath:sql/**/*.xml"));
return sqlSessionFactoryBean.getObject();
}
@Bean(name = "dbMybatisSqlSessionTemplate")
@Primary
public SqlSessionTemplate dbMybatisSqlSessionTemplate(SqlSessionFactory dbMybatisSqlSessionTemplate) throws Exception {
return new SqlSessionTemplate(dbMybatisSqlSessionTemplate);
}
@Bean(name="dbMybatisTransactionManager")
@Primary
public PlatformTransactionManager dbMybatisTransactionManager(@Qualifier("dbMybatisSource") DataSource dbMybatisSource) {
DataSourceTransactionManager transactionManager = new DataSourceTransactionManager();
transactionManager.setDataSource(dbMybatisSource);
return transactionManager;
}
}
@MapperScan(value="com.juntcom.api.mapper.*", sqlSessionFactoryRef="dbMybatisSqlSessionFactory")
@MapperScan -> mapper 클래스를 놓을 클래스 경로가 맞아야 한다.
mapper 라는 패키지 하위에 mapper interface 를 위치 시켜야 mapper 를 스캔한다.
private static final String prefix = "spring.db.datasource.hikari.";
라고 선언부가 application.yml 파일에서 가져오기 위한 prefix 이다. 따로 설정하지 않고, class 파일 내부에서 작성해도 되나, 설정값을 한눈에 보기 위해 application.yml 에 기입하는게 낫다.
("classpath:sql/**/*.xml"));
resources 하위에 sql 폴더 생성 후 그 밑에 xml 파일을 만들자.
경로는 sql/*.xml 로 sql 폴더 바로 밑에 xml 파일 두고 싶은경우는 다음과 같이 하자.
나 같은 경우는 read 폴더 및 writer 폴더를 나누는게 좋아서 /**/ 를 추가했다.
참고
datasource 를 하나만 둘 거면 yml 에 설정값을 추가함으로써 db connection 을 할 수 있지만,
java 파일에서 한 이유는
여러개의 db 를 사용할 경우 multi datasource 를 사용하기 위함이다.
yml 에 db1, db2 라고 추가해 java class 파일을 별도로
class1 {
private static final String prefix = "spring.db1.datasource.hikari.";
...
}
class2 {
private static final String prefix = "spring.db2.datasource.hikari.";
...
}
와 같이 class 를 별도로 두면 된다.
application.yml
spring:
db: #db1 이든 db2 이든 상관없다. java class 의 prefix 의 값과 맞춰주면 된다.
datasource:
hikari:
jdbc-url: jdbc:mysql://localhost:3306?&useSSL=false&characterEncoding=UTF-8&serverTimezone=UTC
username: root
password: 비밀번호
driverClassName: com.mysql.cj.jdbc.Driver
maximum-pool-size: 10
#minimum-idle: 100
max-lifetime: 1800000 #1800000
connection-timeout: 30000 #30000
validation-timeout: 5000
#connection-test-query: SELECT 1
data-source-properties:
cachePrepStmts: true
prepStmtCacheSize: 250
prepStmtCacheSqlLimit: 2048
useServerPrepStmts: true
위까지만 하면 datasource 가 뜨기까지의 설정은 되었으나, mapper 클래스 및 resource 하위에 쿼리가 비어있기 때문에 샘플 하나는 만들어야 어플리케이션이 뜬다.
mapper 클래스
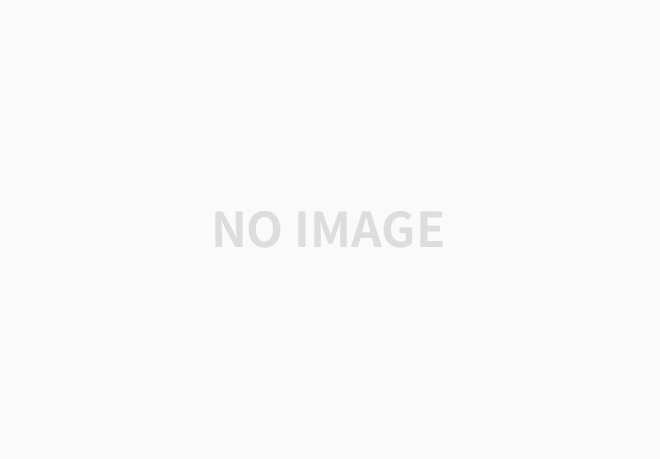
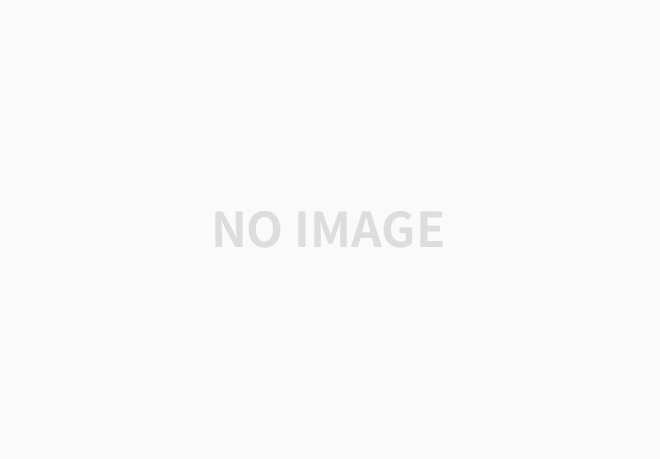
다음과 같이 위치시켜주자. 패키지명을 달리하려면 config 클래스에서 MapperScan 의 값을 변경하자.
@Mapper
public interface ReadMapper {
List<Map<String, Object>> selectCode() throws Exception;
}
. xml 파일
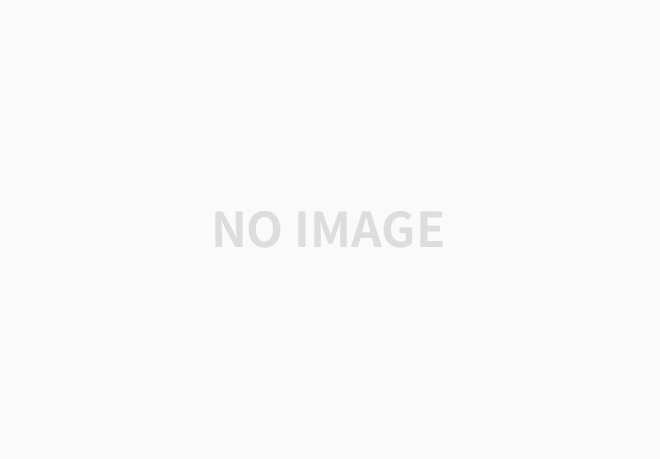
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.juntcom.api.mapper.read.ReadMapper">
<select id="selectCode" resultType="map">
SELECT
*
FROM
TEST
</select>
</mapper>
참고문헌
> https://mybatis.org/spring-boot-starter/mybatis-spring-boot-autoconfigure/ [mybatis spring boot starter 공식문서]
'Spring > spring boot 및 기타' 카테고리의 다른 글
[spring] Mybatis batch upsert 하는 방법(mysql) (0) | 2021.08.11 |
---|---|
[Spring] multi datasource 동적으로 사용 - AbstractRoutingDataSource (0) | 2021.08.03 |
[spring boot] 스프링 firebase database 사용하기 (1) | 2021.05.04 |
[spring boot] 스프링 부트 h2 인메모리 db 시작하기 (0) | 2021.04.27 |
[spring] 스프링 HikariDatasource 속성 값 (0) | 2021.03.31 |